Optimistic Navigation: Creating the Illusion of Instant Navigation
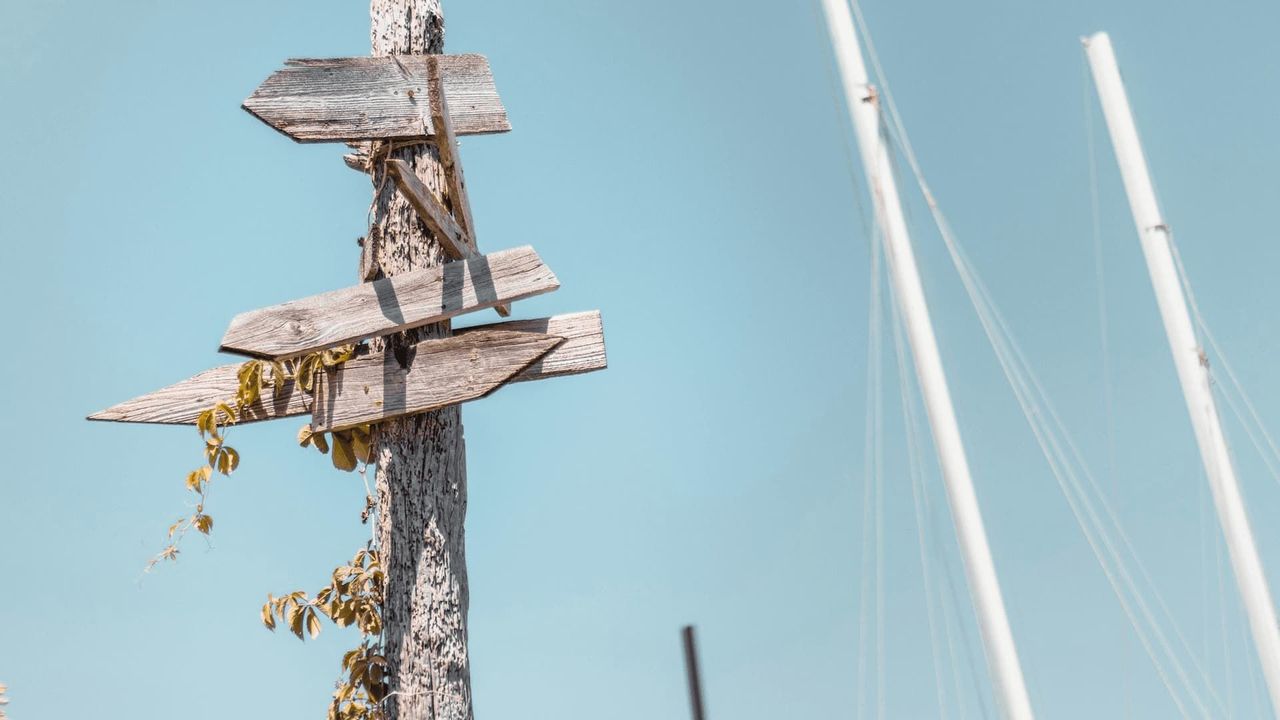
In a world where speed defines digital success, a sluggish website can drive users away. Weak internet connections or overloaded servers often make even modern websites feel slow and unresponsive. Optimistic navigation offers a clever solution: by updating the user interface (UI) optimistically, it masks network delays, making navigation feel faster—or even instantaneous—than it truly is.
The Problem: Slow Navigation and Lack of Response
Picture this: you click a link, and nothing happens for a full second. The screen freezes, offering no sign of progress. It’s not just slow—it feels broken. This is the frustration users face when network conditions falter or servers lag. Check out the demo app below, where I’ve simulated this pain point: every navigation takes a deliberate one-second delay. Try clicking an article card or the “Home” link to experience it yourself.
What are optimistic UI updates?
Optimistic UI updates are all about instant feedback. Instead of waiting for the server, the UI updates immediately, anticipating what the server will return. There are two approaches:
- Render with predicted data: Assume the server’s response will match existing data. For example, if a user clicks to view their profile, show their cached name and photo right away, betting it hasn’t changed.
- Render without predicted data: Display a skeleton version of the page while the real content loads.
If the server responds with an error, these updates roll back smoothly, and the error is handled gracefully.
Optimistic navigation and performance illusion.
Navigation feels lightning-fast when the page is optimistically rendered with predicted data that covers the entire viewport. Users can start interacting with the page immediately, and by the time they scroll to unloaded sections, the actual data might already be there. Even if they spot the loading, engaging with predicted content beats staring at a blank screen. Imagine this as giving the user a "sample portion" before presenting the actual product. See it in action in the demo app below.
Data Recycling: Leveraging What’s Already There
Here’s a trick: the data in a list or card (like a thumbnail, title, and description) often mirrors what’s on the detail page—just expanded. Optimistic navigation can recycle this data for instant rendering. For instance, in a blog app, a list of articles might show titles and snippets. Click through, and that snippet appears instantly on the article page while the rest loads.
If you feel like data from the entity card is not enough, consider increasing it by changing the amount of data returned for that entity listing request. The more data you receive, the more content you can render ahead of time during navigation. Carefully crafted optimistic navigation can hide 5–10 seconds of delays from the user. I achieved such results in that Tanstack-Start blog starter. Navigate through that demo with enabled 3G network throttling to see optimistic navigation in action.
Building trust through consistency
Optimistic navigation delivers consistency: every navigation renders in about 16 milliseconds. It’s seamless, like flipping through a glossy magazine. This reliability builds trust, keeping users engaged and satisfied, even on shaky networks.
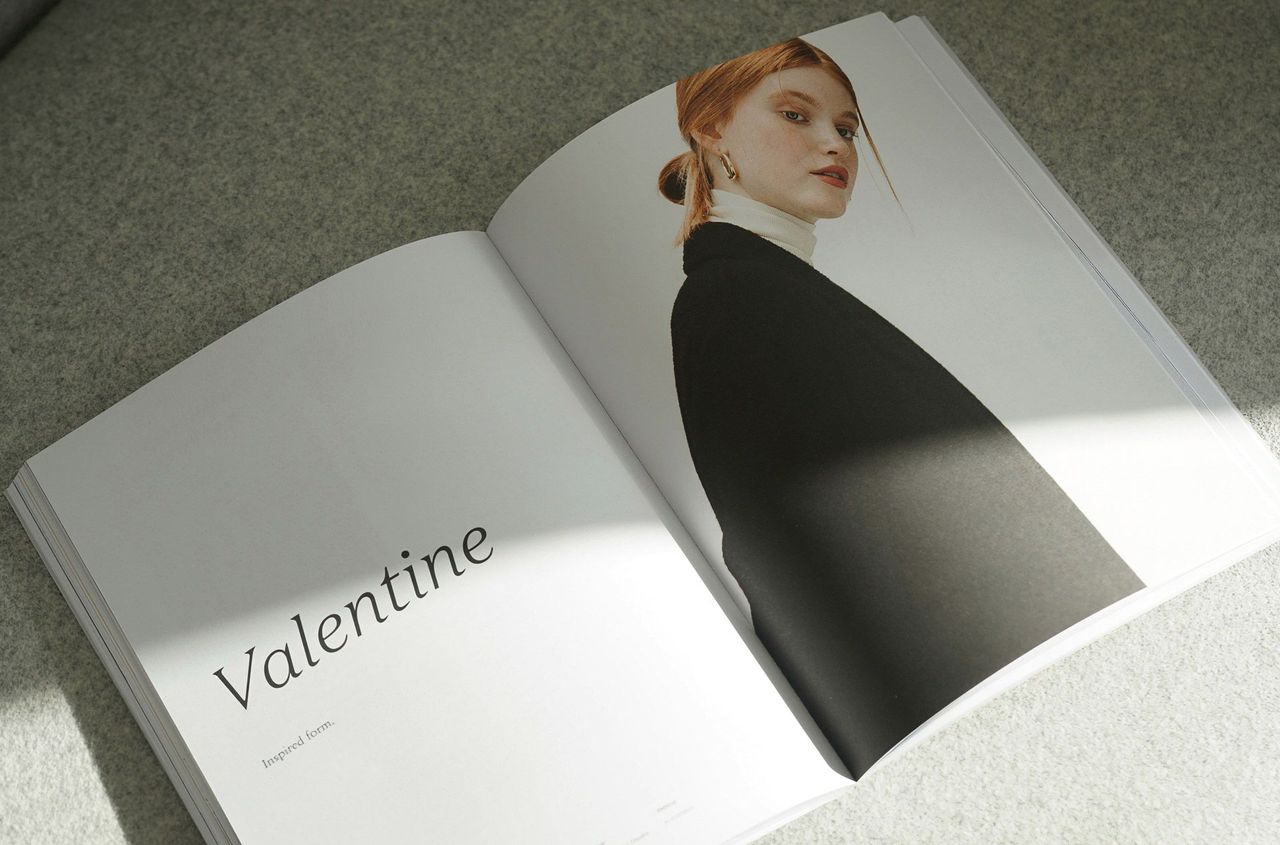
The Downsides of Optimistic Navigation
No solution is flawless. Here are the trade-offs:
- May require additional API calls or database queries.
- RAM Usage: Storing predicted data can increase memory use, though modern browsers manage this efficiently.
- Potential Confusion: If predicted data mismatches the server’s response, the page re-renders.
Prefetching vs. Optimistic Navigation
Prefetching is often used instead of optimistic navigation. Prefetching happens once the link is visible in the viewport or when it is hovered. It significantly reduces perceived load time once the user decides to click on a link.
Pros:
- Easy to use, supports in most routers/meta-frameworks
- Can significantly speed up navigation
Cons:
- Inconsistency: Not always effective for users with slower connections or devices.
- Environmental Impact: Increases CO2 emissions due to more network requests.
- Resource Consumption: Drains battery life, increases internet usage, and hosting costs.
- SEO Penalties: Might negatively affect SEO due to excessive resource use.
- Data Staleness: Preloaded content can become outdated once used for rendering.
Conclusion
Modern web development increasingly bets on pre-downloading content for speed. Optimistic navigation takes a different tack: it conjures the illusion of instant loading, elevating perceived performance and responsiveness. Making navigation feel fast without wasting user or server resources.